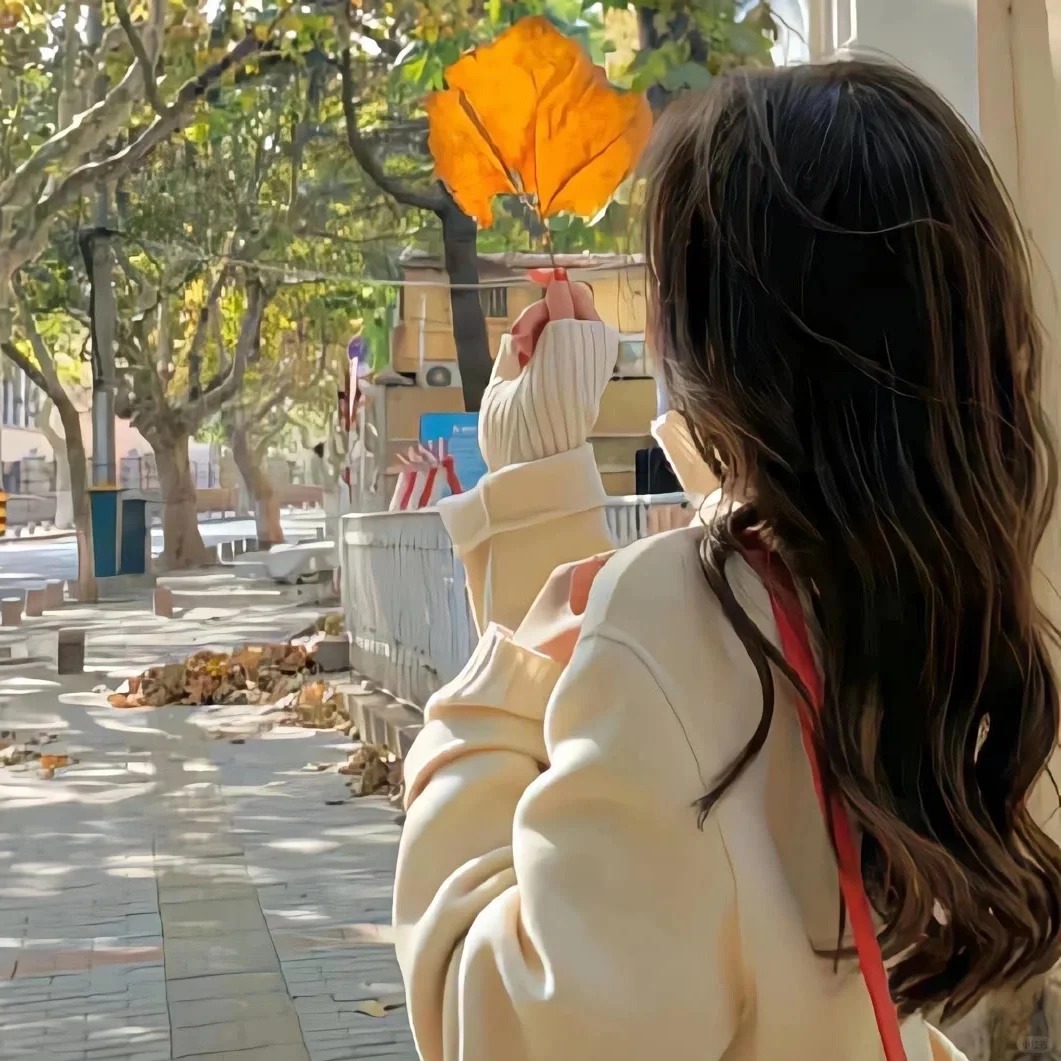
0
el-plus表头筛选表格显示字段
羊羊2024-12-210
order.store.js
在order.store.ts
文件里面定义
import { defineStore } from "pinia";
import { computed, reactive, ref, watch } from "vue";
interface columsInterface {
prop?: string;
label?: string;
width?: number | string;
fixed?: string;
align?: string;
require?: boolean;
isChecked: boolean;
sort?: number;
}
export const useOrderStore = defineStore("orderStore", () => {
// 表格的唯一标识(tableKey)
const tableKey = ref("");
// 存储每个表格的静态列配置
const staticColums = ref<columsInterface[]>([]);
// 用于本地存储的列配置函数
function initColums(tableKey: string, staticColumns: columsInterface[]): columsInterface[] {
const storedColumns = localStorage.getItem(tableKey);
let result: columsInterface[] = [];
if (storedColumns) {
result = JSON.parse(storedColumns);
// 在这里处理列的对比
result = syncColumns(result, staticColumns);
} else {
result = staticColumns;
}
return result;
}
// 对比静态列和本地存储的列,进行增删和状态同步
function syncColumns(storedColumns: columsInterface[], staticColumns: columsInterface[]): columsInterface[] {
// 先删除本地存储中存在但静态列中不存在的列
const updatedColumns = storedColumns.filter((storedColumn) => {
return staticColumns.some((staticColumn) => staticColumn.prop === storedColumn.prop);
});
// 遍历静态列,如果本地存储中没有该列,则添加
staticColumns.forEach((staticColumn) => {
const existing = updatedColumns.find((storedColumn) => storedColumn.prop === staticColumn.prop);
if (!existing) {
updatedColumns.push({ ...staticColumn, isChecked: true }); // 新列默认选中状态为true
}
});
// 返回合并后的列配置
return updatedColumns;
}
// 设置表格的静态列
const setStaticColums = (columns: columsInterface[]) => {
staticColums.value = columns;
// 每次设置静态列后,需要重新初始化列配置
allColums.splice(0, allColums.length, ...initColums(tableKey.value, staticColums.value));
};
// 所有列的状态
const allColums = reactive<columsInterface[]>(initColums(tableKey.value, staticColums.value));
// 当前选中的列
const colums = computed(() => {
return allColums.filter((item) => item.isChecked).sort((item1, item2) => item1.sort - item2.sort);
});
const isIndeterminate = ref(false);
const checkedAll = ref(false);
// 保存列配置到本地存储
const saveColums = () => {
localStorage.setItem(tableKey.value, JSON.stringify(allColums));
};
watch(
() => allColums,
(newVal) => {
if (allColums.length > 0) {
saveColums();
}
checkedAll.value = newVal.every((i) => i.isChecked);
},
{ deep: true, immediate: true }
);
// 全选/全不选
function selectAll(checked: boolean) {
allColums.forEach((item) => {
if (!item.require) {
item.isChecked = checked;
}
});
}
// 设置表格唯一键
const setTableKey = (key: string) => {
tableKey.value = key;
// 刷新数据
allColums.splice(0, allColums.length, ...initColums(tableKey.value, staticColums.value));
};
// 返回给组件使用
return {
colums,
allColums,
selectAll,
checkedAll,
isIndeterminate,
setTableKey,
setStaticColums,
};
});
使用方法
order/index.vue
let orderStaticColums = [
{
label: "订单号",
prop: "prepayid",
width: "200",
isChecked: true,
require: true,
sort: 10,
},
{
label: "平台单号",
prop: "paidanid",
align: "center",
isChecked: true,
require: false,
sort: 20,
},
{
label: "订单类型",
prop: "type",
width: "80",
isChecked: true,
require: true,
sort: 30,
},
{
label: "订单状态",
prop: "status",
width: "160",
isChecked: true,
require: true,
sort: 40,
},
{
label: "订单金额",
prop: "moneyPrice",
width: "120",
isChecked: true,
require: true,
sort: 50,
},
{
label: "订单描述",
prop: "describe",
width: "160",
align: "center",
isChecked: true,
require: false,
sort: 60,
},
{
label: "订单地址",
prop: "address",
width: "260",
align: "center",
isChecked: true,
require: false,
sort: 70,
},
{
label: "下单时间",
prop: "time_create",
width: "160",
align: "center",
isChecked: true,
require: false,
sort: 80,
},
{
label: "接单时间",
prop: "time_pay",
width: "160",
align: "center",
isChecked: true,
require: false,
sort: 90,
},
{
label: "取消时间",
prop: "time_cancel",
width: "160",
align: "center",
isChecked: true,
require: false,
sort: 100,
},
{
label: "订单操作",
fixed: "right",
prop: "utils",
isChecked: true,
require: true,
sort: 110,
},
];
onMounted(() => {
orderStore.setTableKey('cc')
orderStore.setStaticColums(orderStaticColums); // 设置当前表格的静态列
})
order/all.vue
let orderStaticColums = [
{
label: "订单号",
prop: "prepayid",
width: "200",
isChecked: true,
require: true,
sort: 10,
},
{
label: "平台单号",
prop: "paidanid",
align: "center",
isChecked: true,
require: false,
sort: 20,
},
{
label: "订单类型",
prop: "type",
width: "80",
isChecked: true,
require: true,
sort: 30,
},
{
label: "订单状态",
prop: "status",
width: "160",
isChecked: true,
require: true,
sort: 40,
},
{
label: "订单金额",
prop: "moneyPrice",
width: "120",
isChecked: true,
require: true,
sort: 50,
},
{
label: "订单描述",
prop: "describe",
width: "160",
align: "center",
isChecked: true,
require: false,
sort: 60,
},
{
label: "订单",
prop: "describe",
width: "160",
align: "center",
isChecked: true,
require: false,
sort: 60,
},
{
label: "订单地址",
prop: "address",
width: "260",
align: "center",
isChecked: true,
require: false,
sort: 70,
},
{
label: "下单时间",
prop: "time_create",
width: "160",
align: "center",
isChecked: true,
require: false,
sort: 80,
},
{
label: "接单时间",
prop: "time_pay",
width: "160",
align: "center",
isChecked: true,
require: false,
sort: 90,
},
{
label: "取消时间",
prop: "time_cancel",
width: "160",
align: "center",
isChecked: true,
require: false,
sort: 100,
},
{
label: "订单操作",
fixed: "right",
prop: "utils",
isChecked: true,
require: true,
sort: 110,
},
];
onMounted(() => {
orderStore.setTableKey('A')
orderStore.setStaticColums(orderStaticColums)
})
版权声明
本文系作者 @羊羊 转载请注明出处,文中若有转载的以及参考文章地址也需注明。\(^o^)/~
Preview